Stay ahead of the latest in secure coding.
The cyber-threat landscape is constantly shifting and staying ahead of the curve is more important than ever. With a range of videos, blogs, and handy secure coding guidelines, we can help keep you up to date and ready for what comes next.


Our latest secure coding guidelines.
{
"dependencies": {
"foo": "1.0.0 - 2.9999.9999",
"bar": ">=1.0.2 <2.1.2"
}
}
Using Components with Known Vulnerabilities
{
"dependencies": {
"foo": "1.0.0 - 2.9999.9999",
"bar": ">=1.0.2 <2.1.2"
}
}
Most applications make use of large amounts of third-party components. These components provide everything from logging, templating, database access, and more. This makes developing software much easier and saves a lot of time. But they're also made by people, which means some will inevitably contain vulnerabilities. Read the guideline to find out more.
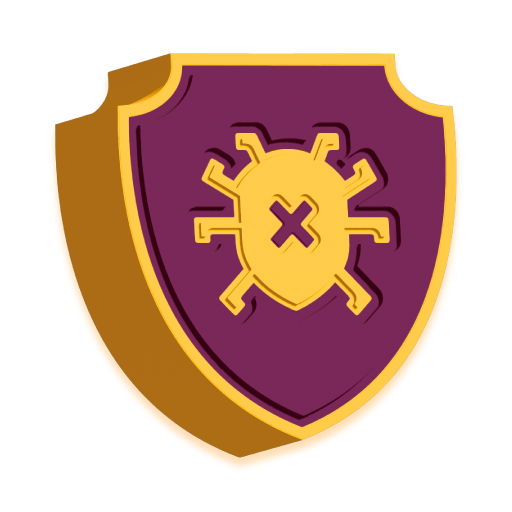
import mysql.connector
db = mysql.connector.connect
#Bad Practice. Avoid this! This is just for learning.
(host="localhost", user="newuser", passwd="pass", db="sample")
cur = db.cursor()
name = raw_input('Enter Name: ')
cur.execute("SELECT * FROM sample_data WHERE Name = '%s';" % name) for row in cur.fetchall(): print(row)
db.close()
SQL Injection
import mysql.connector
db = mysql.connector.connect
#Bad Practice. Avoid this! This is just for learning.
(host="localhost", user="newuser", passwd="pass", db="sample")
cur = db.cursor()
name = raw_input('Enter Name: ')
cur.execute("SELECT * FROM sample_data WHERE Name = '%s';" % name) for row in cur.fetchall(): print(row)
db.close()
SQL injection (SQLi) injects code into SQL statements to attack and gather important information from an application. It is a web security vulnerability. It is the most common technique of hacking that manipulates the database and extracts crucial information from it.
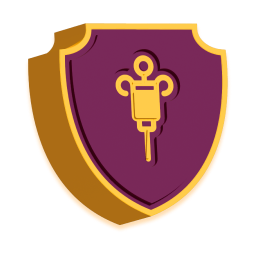
ts
let url = request.params.url;
let response = http.get(url);
let render = response.render();
return render.export();
Server-Side Request Forgery
ts
let url = request.params.url;
let response = http.get(url);
let render = response.render();
return render.export();
Server-Side Request Forgery vulnerabilities occur when a user is able to cause an application to make HTTP requests to an attacker-determined domain. If an application has access to private/internal networks, an attacker could also cause the application to make requests to internal servers. We’ll take a closer look at this with some examples to better understand what it looks like in action in this guideline.

Many frameworks also have a set of endpoints that can be enabled which allows for monitoring of the application, whether that's in a production or test/dev environment. These can include:
Metrics (Prometheus)
Logs
Environment information
Path/Url Mappings
Security Misconfiguration
Many frameworks also have a set of endpoints that can be enabled which allows for monitoring of the application, whether that's in a production or test/dev environment. These can include:
Metrics (Prometheus)
Logs
Environment information
Path/Url Mappings
Security Misconfiguration is somewhat of an umbrella term that covers common vulnerabilities that come into play because of an application’s configuration settings, rather than bad code. It’s a wide-ranging subject and it’s heavily dependent on factors like your technology stack. Often times addressing these issues is something that seems simple, like changing a configuration file or even a single line of code, but the impact and consequences of these vulnerabilities can be severe. Read our guideline to learn more about this vulnerability and how to mitigate it.

Real-world secure coding missions.
Explore our library of public training exercises. These sample SCW Missions give you guided hands-on experience with some offensive secure coding practices in a real-world app simulation.